Table of Contents
本文通过实例介绍如何在 c#中调用 C++编写的 dll。
1 首先用 C++编写一个测试用的 dll
1.1 新建一个 Win32 控制台工程
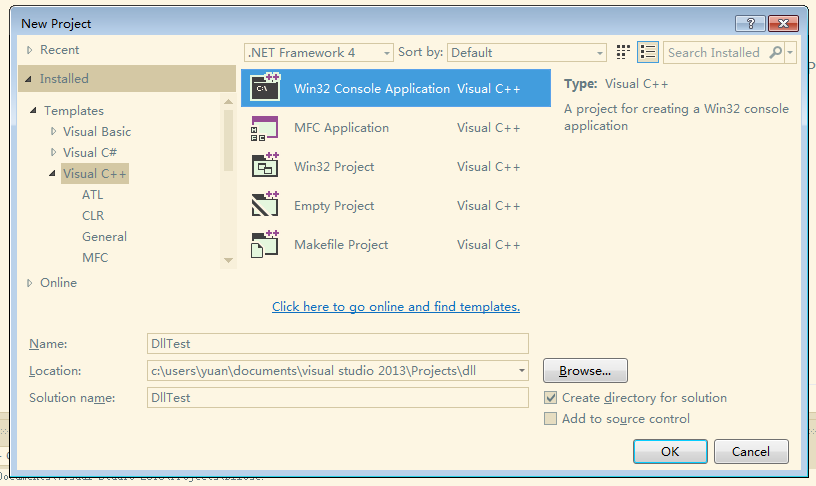
1.2 Application settings 中将应用类型设置为 DLL
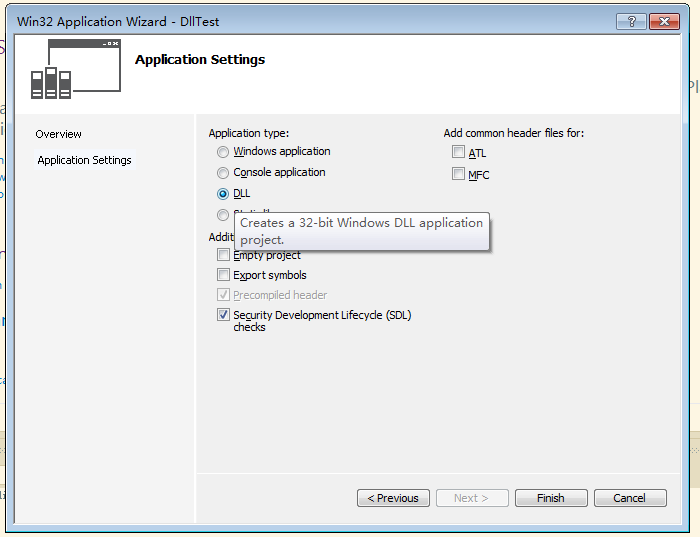
1.3 在.cpp 文件中输入代码
#include "stdafx.h" |
关键的语句是:extern "C" __declspec(dllexport) void __stdcall
如果没有 __stdcall, 程序依然可以执行,但是调试时会报错。
2 C#中新建控制台程序,对 dll 进行测试
2.1 C#代码
using System; |
2.2 将 C++生成的 Dll 文件拷贝至 C#工程的调试目录下,然后就可以运行了
2.3 运行结果
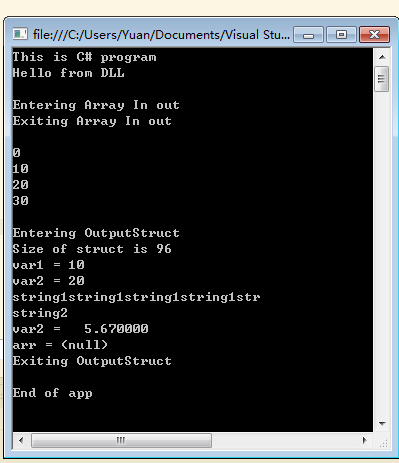
Date:
Created: 2016-09-22 四 17:13